Category Subcategory Dropdown In PHP MySQL Ajax With Example
Dynamic Category and Subcategory Dropdown in PHP MySQL Ajax. In this tutorial, we will show you how to create categories and subcategories in PHP MySQL using Ajax.
Sometimes, you need to select a query in PHP so we create separate tables to display selected subcategories based on selected category dropdowns. But in this tutorial, we will use/learn only a single table use (category and subcategory in one table) for a PHP dynamic fetch data from database in dropdownlist in PHP.
This tutorial will help you step by step how to fetch data from a database in PHP using MySQL on dynamic category and subcategory dropdown list onchange in PHP MySQL using Ajax or populate the second dropdown based on the first dynamic dropdown in PHP. As well as learn, how to fetch data from database in PHP using MySQL.
In this post category subcategory dropdown in PHP MySQL example, we will show subcategory in the dropdown list based on the selected category in PHP using ajax from the database.
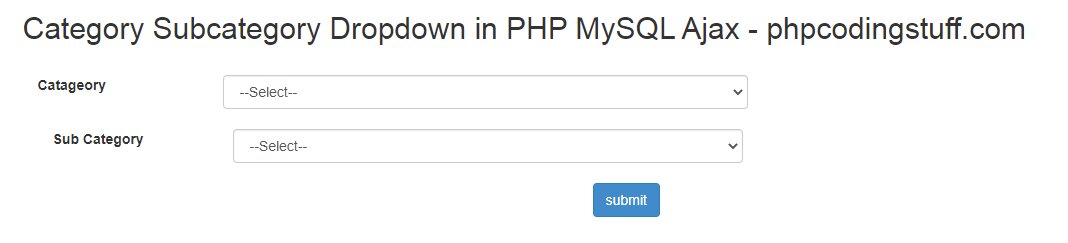
Dynamic Category and Sub-Category Dropdown List in PHP using Ajax
Follow the below simple and easy code display category and subcategory in dropdown list onchange in PHP MySQL using jQuery ajax:
- Step 1: Create Table
- Step 2: Insert Data In Table(Category and SubCategory)
- Step 3: Create DB Connection PHP File
- Step 4: Create Form And Category, SubCategory Dropdown in Form
- Step 5: Get Sub Category in Dropdown List by Category Id
Open your database and run the following SQL query to create categories table into database:
CREATE TABLE `categories` ( `id` int(11) NOT NULL AUTO_INCREMENT, `parent_id` int(11) NOT NULL DEFAULT '0', `category` varchar(255) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
In this step, run the following SQL query to insert category and subcategory into MySQL database in PHP:
INSERT INTO `categories` (`id`, `parent_id`, `category`) VALUES (1, 0, 'General'), (2, 0, 'PHP'), (3, 0, 'HTML'), (4, 7, 'Tables'), (5, 2, 'Functions'), (6, 2, 'Variables'), (7, 3, 'Forms');
In this step, create a file name db.php and update the following code into the db.php file:
This code is used to create a MySQL database connection in your category subcategory dropdown in PHP MySQL project.
<?php $servername='localhost'; $username='root'; $password=''; $dbname = "my_db"; $conn=mysqli_connect($servername,$username,$password,"$dbname"); if(!$conn){ die('Could not Connect MySql Server:' .mysql_error()); } ?>
In this step, create an index.php file and update the below PHP and HTML code into the index.php file.
This HTML code shows the category and subcategory dropdown list. And the PHP and ajax code of this file will dynamically populate subcategories in the dropdown list based on the selected category in the dropdown and fetch data from database in dropdownlist in php.
Now, update the following PHP MySQL ajax and HTML form into index.php file:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <title>Dynamic Category Subcategory Dropdown in PHP MySQL Ajax - phpcodingstuff.com</title> <!-- Fonts --> <link href="https://fonts.googleapis.com/css?family=Nunito:200,600" rel="stylesheet"> <!-- Styles --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css" > <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <style> html, body { background-color: #fff; color: #636b6f; font-family: 'Nunito', sans-serif; font-weight: 200; height: 100vh; margin: 0; } .full-height { height: 100vh; } .flex-center { align-items: center; display: flex; justify-content: center; } .position-ref { position: relative; } .top-right { position: absolute; right: 10px; top: 18px; } .content { text-align: center; } .title { font-size: 84px; } .links > a { color: #636b6f; padding: 0 25px; font-size: 13px; font-weight: 600; letter-spacing: .1rem; text-decoration: none; text-transform: uppercase; } .m-b-md { margin-bottom: 30px; } </style> </head> <body> <div class="container mt-5"> <div class="row justify-content-center"> <div class="col-md-8"> <div class="card"> <div class="card-header"> <h2 class="text-primary">Category Subcategory Dropdown in PHP MySQL Ajax - phpcodingstuff.com</h2> </div> <div class="card-body"> <form> <div class="form-group"> <label for="CATEGORY-DROPDOWN">Category</label> <select class="form-control" id="category-dropdown"> <option value="">Select Category</option> <?php require_once "db.php"; $result = mysqli_query($conn,"SELECT * FROM categories where parent_id = 0"); while($row = mysqli_fetch_array($result)) { ?> <option value="<?php echo $row['id'];?>"><?php echo $row["category"];?></option> <?php } ?> </select> </div> <div class="form-group"> <label for="SUBCATEGORY">Sub Category</label> <select class="form-control" id="sub-category-dropdown"> </select> </div> </form> </div> </div> </div> </div> </div> <script> $(document).ready(function() { $('#category-dropdown').on('change', function() { var category_id = this.value; $.ajax({ url: "fetch-subcategory-by-category.php", type: "POST", data: { category_id: category_id }, cache: false, success: function(result) { $("#sub-category-dropdown").html(result); } }); }); }); </script> </body> </html>
Now, create a new PHP file name fetch-subcategory-by-category.php. how to fetch data from database in PHP using MySql in This file code will fetch and show a subcategory (second dropdown) based on the selected category (previous dropdown selection) in PHP. We learn dynamic dropdown in PHP.
So, update sub-categories following PHP and HTML code into the fetch-subcategory-by-category.php file:
<?php require_once "db.php"; $category_id = $_POST["category_id"]; $result = mysqli_query($conn,"SELECT * FROM categories where parent_id = $category_id"); ?> <option value="">Select SubCategory</option> <?php while($row = mysqli_fetch_array($result)) { ?> <option value="<?php echo $row["id"];?>"><?php echo $row["category"];?></option> <?php } ?>
In this tutorial, you have learned how to create categories and subcategories in PHP MySQL. a dropdown list based on the selected category in PHP MySQL using Ajax.
I hope it can help you...
Thank you for this code. Its working! But…how can I edit and update it using ajax. Any help would be highly appreciated. Thank you