File Upload With Multer In Node.js And Express
In this tutorial, we are going to learn how to upload images on the server with multer and express in Node.js. File upload is a common operation for any project. Node.js with the Express Web Framework and the multer Library, this tutorial we adding a file upload feature to your app is very easy. You will learn in this tutorial to make you comfortable in building apps that can easily handle any file uploads.
We will be covering the following topics:
- What is Multer?
- Project Setup
- Adding Multer
- Disk Storage
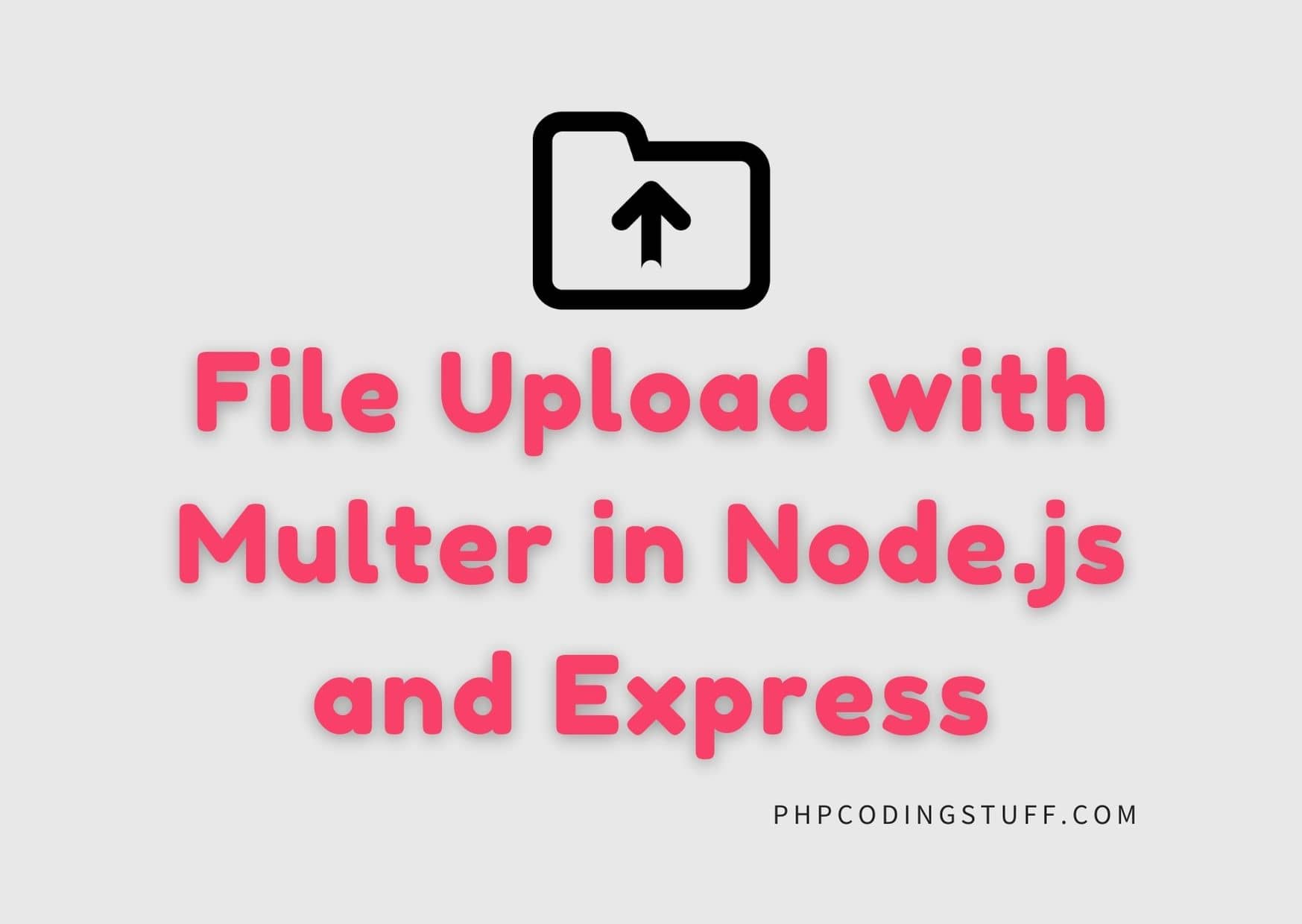
What is Multer?
Multer is a node.js middleware for handling multipart/form-data
, which is primarily used for uploading files. It is written on top of the busboy for maximum efficiency.
NOTE: Multer will not process any form which is not multipart (multipart/form-data)
.
Project Setup
First of all, We Create a directory for the project and give it any name. write the following command:
npm init -y
Adding Multer
Before using multer, we have to install it using npm.
npm install multer --save
Now we will now load multer in the app.js file using the require() method. The following code will go in the app.js file.
const multer = require('multer'); const upload = multer({dest:'uploads/'}).single("demo_image");
Here we are making a post request method="POST" and action="/image" in form tag.
The following code will go in app.js:
app.post("/image", (req, res) => { upload(req, res, (err) => { if(err) { res.status(400).send("Something went wrong!"); } res.send(req.file); }); });
Disk Storage
This Step disk storage engine gives you full control over storing files to disk. We will create a storage object using the diskStorage() method.
The following code will go in app.js:
var storage = multer.diskStorage({ destination: function(req, file, cb) { cb(null, './uploads'); }, filename: function (req, file, cb) { cb(null , file.originalname); } });
Here, there are two properties, destination, and filename. They both are functions.
Destination - It can also be given as a string (e.g. './upload'). If you did not give any destination is given, the operating system's default directory for temporary files is used. Mandatory to create a directory when you are using destination as a function of your project.
Filename - It is used to determine what the file should be named inside the folder in your project. If you don't provide any filename, each file will be given a random name without any file extension. The file object and a callback function (here, CB is callback function). The 2 arguments to CB are: We learn using Multer and Express in Node.js
- null - as we don’t want to show any error.
- file.originalname - here, we have used the same name of the file as they were uploaded. You can use any name of your choice in this file name.
Now let’s modify the variable a little bit.
// In app.js var upload = multer({ storage: storage }).single("demo_image");
I hope it can help you...
Leave a Reply